Posted: November 26th, 2013 | Author: gerdsen | Filed under: arduino, microcontroller, rfduino, wireless | Tags: arduino, microcontroller, rfduino | No Comments »
I got my RFDuino in today (http://www.rfduino.com/), if you aren’t familiar with it don’t worry. The RFDuino is a scaled down Arduino-clone with wireless capabilities (Bluetooth, specifically 4.0) built directly on the board. The project started as a Kickstarter (http://www.kickstarter.com/projects/1608192864/rfduino-iphone-bluetooth-40-arduino-compatible-boa) and has since been funded and deliveries are starting to arrive to earlier backers.
So I got my RFDuino in the mail and am pretty excited to give it a whirl. In addition to the RFDuino, the backing level I was at afforded me a Relay Shield as well as a RGB Push Button shield.
I will continue to update this post as thing progress with any thing I may feel is of help to others.
Stay tuned.
Posted: October 15th, 2013 | Author: gerdsen | Filed under: c#, experimental | Tags: AJAX, ASP, C#, MVC | No Comments »
It’s been a while since I’ve made a post, mostly because I’ve been busy and mostly because I usually post information I feel will be helpful to others. Today I want to share a snippet of code that I put together because I was unable to track down the answer I was looking for.
Currently I am in a long term project that is primarily based ASP.NET MVC3 and with that comes troubles of its own. The trouble I was having was I wanted to create an HTML BUTTON that behaved much like MVC’s Ajax.ActionLink does. I wanted to be able to specifiy some button content as well as AjaxOptions for Unobtrusive parsing. I looked on the web, no luck. So I ventured out and created my own extension that I hope you find worthy.
C# (Place in new or existing file)
public static MvcHtmlString ActionButton(this AjaxHelper ajaxHelper,
string buttonText,
string iconSrc,
AjaxOptions ajaxOptions,
object routeValues = null,
object htmlAttributes = null,
object iconAttributes = null)
{
UrlHelper urlHelper = new UrlHelper(ajaxHelper.ViewContext.RequestContext);
RouteValueDictionary htmlAttributeDictionary =
HtmlHelper.AnonymousObjectToHtmlAttributes(htmlAttributes);
string imgUrl = urlHelper.Content(iconSrc);
//Build icon for use in button
TagBuilder buttonIcon = new TagBuilder("img");
buttonIcon.MergeAttribute("src", imgUrl);
buttonIcon.MergeAttributes(new RouteValueDictionary(iconAttributes));
//Text
TagBuilder text = new TagBuilder("span") { InnerHtml = buttonText };
//Build Button and place text and icon inside
TagBuilder button = new TagBuilder("button");
button.MergeAttributes(ajaxOptions.ToUnobtrusiveHtmlAttributes());
button.MergeAttributes(htmlAttributeDictionary);
button.InnerHtml = buttonIcon.ToString(TagRenderMode.SelfClosing) + text.ToString();
return MvcHtmlString.Create(button.ToString());
}
You can of course overload or customize this extension method to suit your needs (remove routeValues, htmlAttributes, etc…), we actually have a few variants (icon, no icon) for use in our system for different calls but I wanted to show you a full capability call.
We are not out of the woods just yet, Microsoft’s Unobtrusive AJAX support library for jQuery does not attach to Buttons! They support attaching to A, Form, INPUT[type=image] and Submit but not to regular ole Button. So you are going to need to add a little binding in there to get you hooked up. Copy and paste the following.
JavaScript (Place in your Unobtrusive jQuery Library File)
$("button[data-ajax=true]").live("click", function (evt) {
evt.preventDefault();
if (!$(this).attr("disabled")) {
asyncRequest(this, {
url: this.href,
type: "GET",
data: []
});
}
});
Making the call.
Now that you are all set you can use the following in your code in your View to return unobtrusive BUTTONS with AJAX bindings, below is a sample call.
@Ajax.ActionButton("Button Text", "/Images/x_sm.png", new AjaxOptions
{
Url = Url.Action("Add"),
UpdateTargetId = "Details",
OnComplete = "javascript: weAreDone();",
InsertionMode = InsertionMode.Replace,
OnSuccess = "javascript: hooray();"
},
null,
new { id = "btn1"})
That’s it. Now you have an unobtrusive button in MVC3. Get to styling.
I hope this helps you. I think in the future I will allow for iconAttributes to allow for height/width options. Maybe IDK, I might just leave it all up to CSS.
HTML Output from above call:
<button id="btn1" data-ajax-update="#Details" data-ajax-success="javascript:
hooray();" data-ajax-mode="replace" data-ajax="true" data-ajax-url=
"/Assignments/Assignments/Add" data-ajax-complete="javascript: weAreDone();">
<img src="/Images/x_sm.png" alt="" />
<span>Button Text</span>
</button>
Posted: September 15th, 2011 | Author: gerdsen | Filed under: experimental, mobile, wireless | 3 Comments »
I currently have a project in the works that incorporates the Telit862 Quad GPRS module. After having some issues with signal quality in my area I decided it would be good for me to know the current signal strength given there is no visible display for this on the module. Given this fact I decided to incorporate a meter into my application and after a little research I found some articles that explained how AT&T classifies signal strength groups into their now famous “bars”.
Reading CSQ Value from serial
On the Telit module you can issue the AT+CSQ command to have it return the rssi value (http://en.wikipedia.org/wiki/Received_signal_strength_indication). The rssi value is important because it will be used to determine signal strength.
Using a serial connection you can connect to your Telit GPRS module and issue the AT+CSQ command. I am using minicom for this purpose however there might be other applications for your setup.
Anywho after launching minicom and issuing the AT+CSQ command into the prompt my window looks like this.
Welcome to minicom 2.4
OPTIONS: I18n
Compiled on Sep 7 2010, 01:26:06.
Port /dev/ttyS1
Press CTRL-A Z for help on special keys
AT+CSQ
+CSQ 16, 0
You can see the AT+CSQ command returned “+CSQ rssi, ber“, now this doesn’t help us a whole lot right off the bat as there is still some more processing that needs to be done. What we are looking for is the rssi value in this return; it will be used to convert into dBm. dBm is a power ratio in decibels and it is used to judge quality of reception in wireless comm. Well what’s the “ber” for? Don’t worry about it as you won’t necessarily need it we aren’t that sweet and tight.
Strip all unnecessary string information from return
You will need to be able to read this return from serial port using whichever language you desire. Once you read back “+CSQ rssi,ber” you will need to use some string functions to strip away the unneccesary “+CSQ” and “,ber” leaving you with just the rssi integer, but I’ll leave that part up to you.
Translate rssi to dBm
Telit states that it’s rssi values correspond into dBm as following:
0 – (-113dBm or less)
1 – (-111dBm)
2 thru 30 – (-109 dBm thru -53dBm, which equates to about a +2 dBm per rssi step)
31 – (-51 dBm or greater)
99 – (signal unknown)
Ok so this might be confusing but it’s not all that difficult. Telit states that 2-30 rssi range is equal to -109 dBm thru -53 dBm or steps of 2 dBm for each rssi. Note: We know that when our Telit sends back 1 as our rssi value that it is -111dBm, well that’s probably going to be 0 bars and 99 is an unknown signal according to Telit.
Again so for every rssi value our dBm increases 2. So we get the following…
2 rssi = -109 dBM
3 rssi = -107 dBm
4 rssi = -105 dBm
5 rssi = -102 dBm
…
30 rssi = -55 dBm
31 rssi = -53 dBm
Now that we know what each rssi is equal to we can move forward.
AT&T Bars Formula
According to a blog I found on the internet this guy states that he believes AT&T to categorize their “bars” the following way based on dBm:
5 Bars / -75 dBm or greater
4 Bars / -83 to -74 dBm
3 Bars / -95 to -82 dBm
2 Bars / -105 to -94 dBm
1 Bar / -110 to -104 dBm
0 Bars / -111 or less
Ok great but what the shit does that do for me? Well we now know how to bracket our values into bars to get an easy to read signal meter.
Conclusion
If you do the legwork and convert the rssi into dBm using the two step method you will find out the following:
If rssi is equal to 1 you have 0 Bars
If rssi is greater than 1 and less than 6 you have 1 Bar
If rssi is greater than or equal to 6 and less than 10 you have 2 Bars
If rssi is greater than or equal to 10 and less than 15 you have 3 Bars
If rssi is greater than or equal to 15 and less than 20 you have 4 Bars
If rssi is greater than or equal to 20 but not equal to 99 you have 5 Bars
Remember RSSI of 99 is an unknown signal as stated by Telit.
<(x_-)>
Posted: September 1st, 2011 | Author: gerdsen | Filed under: c#, mobile | 2 Comments »
So I got bored the other evening and decided to create an application for my Windows Phone. I decided I wanted to know how long my phone had been on and set out to create an uptime application. There was another app on the market but I didn’t like the look of it (who cares? I do.) so I opened up Visual Studio Express and nailed it out.
Here is the final result.

Splash Screen with Logo
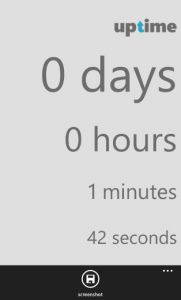
Application Page
Posted: July 27th, 2011 | Author: gerdsen | Filed under: experimental, ramblings | Tags: hacks | No Comments »
I have an old development machine at home that still runs XP. Shoot me. The damn thing works fine and I’m not about to drop the money to upgrade the OS and hardware. I do from time to time like to use it for things it’s not designed for. I recently got an invitation to update my Windows Phone to the latest release of Mango. Well after reading the instructions I noticed I had to install the Windows Phone SDK 7.1, requirements are Vista or Windows 7. Problem.
Solution I found for this was the following:
1. Download the Windows Phone Developer Tools RTW from Microsoft Download Center
2. Extract the downloaded package by running vm_web.exe /x
3. Go to the folder of the extracted package & open the file baseline.dat in any text editor
4. Look for the ‘gencomp7788’ section and change this two entries from 1 to 0:
* InstallOnLHS=0
* InstallOnWinXP=0
5. Save the file baseline.dat
6. Run setup.exe /web
Thanks to Red Frogfish for this information. Hope this helps others.
Posted: April 12th, 2011 | Author: gerdsen | Filed under: ramblings, wireless | Tags: gsm, sarcasm, slow | No Comments »
I have been testing some web snippets with the Telit GM862 module lately, it’s incorporated into a project that I am working on for a client and while ironing out some connectivity issues and yadda-yadda blah blah blah, whatever Joe Rogan. Anyway I just wanted to share some blazing ping times I was getting with the module; ever wonder why your browser seems a bit sluggish to get moving on your phone?
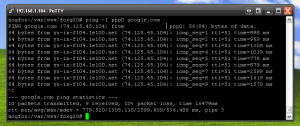
Yes. I still use the Bliss wallpaper and XP.
<(x_-)>
Posted: April 8th, 2011 | Author: gerdsen | Filed under: php | Tags: id3, php | 2 Comments »
The other day a co-worker faced the issue of determining the total duration of multiple mp3 and mp4 files spread across an arbitrary number of directories on the file server. Now we could’ve opened a group of mp3s into Media Player or the like and determined the total duration of that playlist but I couldn’t quickly think of a way to do this for mp4 and right clicking for the file properties was out of the question. Given the fact that this task would become more regular than just a one-time gig I decided to set out and create a script to automate this process. Since I am in bed with PHP I figured I’d go ahead and write this script using just that and run from command prompt.
You will need to download the terrific getID3 class from http://www.getid3.com, this will enable you to perform the necessary file functions required to extract ID3 tags. Once you have downloaded you will need to extract to your working directory from which we will be running our script. By default it will be in a getID3 folder so we will use this for our example.
Ok so let’s make some kludge, open up Notepad or whichever IDE you are comfortable with and start this schniz.
Include ID3 and create instance of ID3
//Require our newly downloaded getid3, main file is getid3.php.
//Under the Linux Environment you would use forward slashes,
//e.g. require_once('/getid3/getid3.php');
require_once('\\getid3\\getid3.php');
//Create instance of ID3
$getID3 = new getID3;
Since I am running this script from the command prompt I am going to ask the user to input the directory they wish to search.
Prompt user for directory they wish to search
//Prompt user for directory location and store in $directoryToSearch
echo "Input the directory to search (C:\\\BLAH\\\FOO):";
$directoryToSearch = trim(fgets(STDIN));
We will declare a variable that will contain our desired file type, this will be used in a check later on in the script. Also we will declare a variable to hold our total duration.
File type and Time variable declaration
//Declare file type we wish to find
$fileType = "mp3";
$totalTime = 0;
We will read through the inputted directory and grab each file one by one. Once we grab a file we will utilize the analyze function of ID3
Traverse Directory and analyze file if found, placing tags into array
//Check to make sure directory is valid
if(is_dir($directoryToSearch)){
//Directory is valid, open it.
$dir = opendir($directoryToSearch);
//Loop through each file in the directory
while (($file = readdir($dir)) !== false) {
if (is_file($fileName)) {
$fileName = realpath($directoryToSearch.'/'.$file);
$getID3->analyze($fileName);
//Put tag information into an array for output
getid3_lib::CopyTagsToComments($currentFile);
There are many different tags you can retrieve using ID3, I am just interested in the file type and the total duration so we will use $array['playtime_seconds']. For more information on what information is available review the structure.txt file or view them here.
Here will double check that this file is our desired file format and if so accumulate the duration.
Check for file type match and accumulate time
//Check to see if the file matches types declared above
//if so accumulate total.
if($currentFile['fileformat'] == $fileType){
//Accumulate
$totalTime = $totalTime + $currentFile['playtime_seconds'];
}
}
}
Now remember this script was made to traverse the Windows Environment; so if you are compiling on Linux make sure you correct your slashes. Also the $directoryToSearch is input at the console, you may wish to change this to a static variable or maybe even a form field if you are migrating this to a web application.
In the real world version of this script I included file operations to output information to a tab delimited file for use in Excel. You may want to add some of this functionality to your script as well or maybe even store to a DBMS of your choice.
<(x_-)>
Posted: April 8th, 2011 | Author: gerdsen | Filed under: ramblings | Tags: i7, oops, processor, random | No Comments »
So we tracked down the problem to the computer that apparently had a mind of its’ own, shutting down at random times throughout the day. While the obvious answer anyone would guess is overheating, supposedly the i7 should scale down it’s speed if heat becomes an issue it cannot overcome so this was thrown out of the window. But what we didn’t know was that the i7 cannot scale itself down when it doesn’t even have a heat sink touching it to help keep it cool.
Hide your wife, hide your kids and go check to make sure your heat sink is properly attached before burning (<— like that pun?) through some 3D renders.
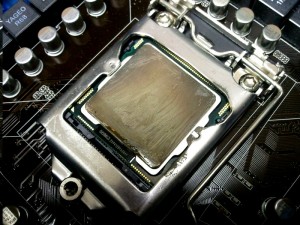
i7 showing some skin, errrr brown skin.
*No processors were harmed in this article.
<(x_-)>
Posted: April 8th, 2011 | Author: gerdsen | Filed under: linux, wireless | Tags: linux, ppp, telit | No Comments »
I thought I would share my experience with connecting to AT&T’s wireless network via Telit GM862 radio over PPP. It is safe to assume this will work for anyone else using another form of GSM device on AT&T’s network.
While there are a couple of posts and a couple of threads that explain what you will need to do to achieve this I decide that it would just be best if I flat out pasted my settings files and was verbatim about the entire situation as it was a headache for me to nail it down.
First step is to ensure you have PPP installed, if you do not you will need to download.
Install PPP
root:~# apt-get update
root:~# apt-get install ppp
Next navigate to your provider file, it should be located in /etc/ppp/peers/provider and edit it to contain the proper information. I have changed the serial device to reflect the proper device, mine was /dev/ttyS1 you might find yours to be mounted elsewhere. Also I have changed the call string to *99***1#”
/etc/ppp/peers/provider contents
# This is the default configuration used by pon(1) and poff(1).
# See the manual page pppd(8) for information on all the options.
# MUST CHANGE: replace myusername@realm with the PPP login name given to
# your by your provider.
# There should be a matching entry with the password in /etc/ppp/pap-secrets
# and/or /etc/ppp/chap-secrets.
user "WAP@CINGULARGPRS.COM"
# MUST CHANGE: replace ******** with the phone number of your provider.
# The /etc/chatscripts/pap chat script may be modified to change the
# modem initialization string.
connect "/usr/sbin/chat -v -f /etc/chatscripts/pap -T *99***1#"
# Serial device to which the modem is connected.
/dev/ttyS1
# Speed of the serial line.
115200
# Assumes that your IP address is allocated dynamically by the ISP.
noipdefault
# Try to get the name server addresses from the ISP.
usepeerdns
# Use this connection as the default route.
defaultroute
# Makes pppd "dial again" when the connection is lost.
persist
# Do not ask the remote to authenticate.
noauth
Next let’s edit our pap file which is located in /etc/chatscripts/pap. Copy and paste, overwriting what is currently in there with what you see below.
/etc/chatscripts/pap contents
# You can use this script unmodified to connect to sites which allow
# authentication via PAP, CHAP and similar protocols.
# This script can be shared among different pppd peer configurations.
# To use it, add something like this to your /etc/ppp/peers/ file:
#
# connect "/usr/sbin/chat -v -f /etc/chatscripts/pap -T PHONE-NUMBER"
# user YOUR-USERNAME-IN-PAP-SECRETS
# noauth
# Uncomment the following line to see the connect speed.
# It will be logged to stderr or to the file specified with the -r chat option.
#REPORT CONNECT
TIMEOUT 60
ABORT BUSY
ABORT VOICE
ABORT "ERROR"
ABORT "NO CARRIER"
ABORT "NO DIALTONE"
ABORT "NO DIAL TONE"
"" ATZ
OK AT+CGDCONT=1,"IP","WAP.CINGULAR"
#,"0.0.0.0",0,0
OK ATD*99***1#
CONNECT
Next we are going to edit our pap-secrets file which can be found in/etc/ppp/pap-secrets. Here we are going to make sure our user has access to pppd, I am running on an embedded linux board that automatically boots to root so I have documented him out so I have access. Also we are going to add our user and password that we inserted into provider file. This must match for authentication to work.
/etc/ppp/pap-secrets contents
#
# /etc/ppp/pap-secrets
#
# This is a pap-secrets file to be used with the AUTO_PPP function of
# mgetty. mgetty-0.99 is preconfigured to startup pppd with the login option
# which will cause pppd to consult /etc/passwd (and /etc/shadow in turn)
# after a user has passed this file. Don't be disturbed therefore by the fact
# that this file defines logins with any password for users. /etc/passwd
# (again, /etc/shadow, too) will catch passwd mismatches.
#
# This file should block ALL users that should not be able to do AUTO_PPP.
# AUTO_PPP bypasses the usual login program so it's necessary to list all
# system userids with regular passwords here.
#
# ATTENTION: The definitions here can allow users to login without a
# password if you don't use the login option of pppd! The mgetty Debian
# package already provides this option; make sure you don't change that.
# INBOUND connections
# Every regular user can use PPP and has to use passwords from /etc/passwd
* hostname "" *
# UserIDs that cannot use PPP at all. Check your /etc/passwd and add any
# other accounts that should not be able to use pppd!
guest hostname "*" -
master hostname "*" -
#root hostname "*" -
support hostname "*" -
stats hostname "*" -
# OUTBOUND connections
# Here you should add your userid password to connect to your providers via
# PAP. The * means that the password is to be used for ANY host you connect
# to. Thus you do not have to worry about the foreign machine name. Just
# replace password with your password.
# If you have different providers with different passwords then you better
# remove the following line.
'WAP@CINGULARGRPS.COM' * 'CINGULAR1' *
Lastly we are going to edit our chap-secrets file, which can be found in the same directory as above. I have removed all other information found in here and added only the AT&T information necessary.
/etc/ppp/chap-secrets contents
# Secrets for authentication using CHAP
# client server secret IP addresses
WAP@CINGULARGPRS.COM WAP.CINGULAR CINGULAR1
Now you are ready to rock and roll on AT&T’s 3G network using your GSM device. To initiate the connect you will issue the pon command from the terminal. To kill the connection you will issue the poff command from the terminal.
By typing plog you can see the last entries in the ppp log. This will help you to verify that it is working, or also to tell if there is an error occuring.
starting ppp and checking the ppp log
root:~# pon
root:~# plog
Apr 5 12:37:33 localhost pppd[1289]: pppd 2.4.4 started by root, uid 0
Apr 5 12:37:34 localhost chat[1290]: timeout set to 60 seconds
Apr 5 12:37:34 localhost chat[1290]: abort on (BUSY)
Apr 5 12:37:34 localhost chat[1290]: abort on (VOICE)
Apr 5 12:37:34 localhost chat[1290]: abort on (ERROR)
Apr 5 12:37:34 localhost chat[1290]: abort on (NO CARRIER)
Apr 5 12:37:34 localhost chat[1290]: abort on (NO DIALTONE)
Apr 5 12:37:34 localhost chat[1290]: abort on (NO DIAL TONE)
Apr 5 12:37:34 localhost chat[1290]: send (ATZ^M)
Apr 5 12:37:34 localhost chat[1290]: expect (OK)
Apr 5 12:37:34 localhost chat[1290]: ATZ^M^M
Apr 5 12:37:34 localhost chat[1290]: OK
Apr 5 12:37:34 localhost chat[1290]: -- got it
Apr 5 12:37:34 localhost chat[1290]: send (AT+CGDCONT=1,"IP","wap.cingular","0.0.0.0",0,0^M)
Apr 5 12:37:35 localhost chat[1290]: expect (OK)
Apr 5 12:37:35 localhost chat[1290]: ^M
Apr 5 12:37:35 localhost chat[1290]: AT+CGDCONT=1,"IP","wap.cingular","0.0.0.0",0,0^M^M
Apr 5 12:37:35 localhost chat[1290]: OK
Apr 5 12:37:35 localhost chat[1290]: -- got it
Apr 5 12:37:35 localhost chat[1290]: send (ATD*99***1#^M)
Apr 5 12:37:35 localhost chat[1290]: expect (CONNECT)
Apr 5 12:37:35 localhost chat[1290]: ^M
Apr 5 12:37:35 localhost chat[1290]: ATD*99***1#^M^M
Apr 5 12:37:35 localhost chat[1290]: CONNECT
Apr 5 12:37:35 localhost chat[1290]: -- got it
Apr 5 12:37:35 localhost chat[1290]: send (^M)
Apr 5 12:37:35 localhost pppd[1289]: Serial connection established.
Apr 5 12:37:35 localhost pppd[1289]: Using interface ppp0
Apr 5 12:37:35 localhost pppd[1289]: Connect: ppp0 <--> /dev/ttyS1
Apr 5 12:37:36 localhost pppd[1289]: Remote message: Welcome!
Apr 5 12:37:36 localhost pppd[1289]: PAP authentication succeeded
Apr 5 12:37:40 localhost pppd[1289]: local IP address 10.11.53.134
Apr 5 12:37:40 localhost pppd[1289]: remote IP address 192.168.0.129
Apr 5 12:37:40 localhost pppd[1289]: primary DNS address 68.105.28.11
Apr 5 12:37:40 localhost pppd[1289]: secondary DNS address 68.105.29.11
You can also issue ifconfig to list all your network interfaces to double check that your ppp0 interface has received the proper addressing.
Remember, to kill this connection simply type poff at the terminal.
Side notes:
Some of you may have problems connecting to web resources and other web objects once establishing a connection even though you have the proper information. This could be due to information in your route, to ensure GPRS routing make sure that you don’t have any default gateways set up in your route. To find out type route in the terminal.
Removing default gateway
root:~# route
Kernel IP routing table
Destination Gateway Genmask Flags Metric R ef Use Iface
192.168.1.0 * 255.255.255.0 U 0 0 0 eth0
default 191.168.0.1 0.0.0.0 UG 0 0 0 eth0
root:~# route del default gw 192.168.0.1 eth0
root:~#
<(x_-)>